lab RISC-V
L1¶
Problem: (N-bit)two’s complement convert to decimal
In two’s complement notation the highest-order bit represents -2^(n-1) instead of +2^(n-1)
Notice 1: high-order bit of the N-bit repre has negative weight.
Notice 1.1: all numbers with MSB of 1 are negative
Notice 1.2: most negative/positive number, range
- Unsigned: 2 ** N - 1;
- 2’s complement: 2 ** (N-1) - 1
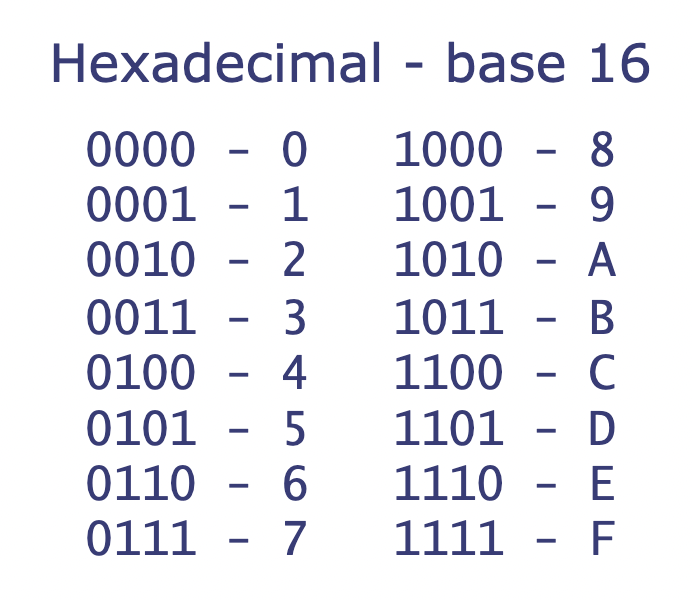
Problem: addition
reason the correctness of the result by performing the addition(mod N) and compare the sign bit of the result to the sign bit of one of the addends when the sign bits of the addends are the same.
Notice 2: overflow when it is needed to drop extra digits.
remark: Note that if you are only given a sequence of bits, you don’t actually know what number they
represent. You also need be told how to interpret those bits: as an unsigned number or as a
signed number stored in two’s complement representation.
try to prove:
Example: Given an unsigned n-bit binary integer = 𝒃𝒏*𝟏… 𝒃𝟏𝒃𝟎 , prove that 𝒗 is a multiple of 4 if and
only if 𝒃𝟎= 𝟎 and 𝒃𝟏= 𝟎.
L2¶
Fact: Logical shift correspond to (left-shift) multiplication by 2, (right-shift) integer division by 2.
Arithmetic shift is something related to 2’s-complement representation of signed numbers. In this representation, the sign is the leftmost bit, then arithmetic shift preserves the sign (this is called sign extension).
-
Load/store word instructions are related to address
sw x4, 4(x3): if register x3 contains 0x2000 and register x4 contains 0x3, the instruction “sw x4, 4(x3)” will store the value 0x3 into the memory location 0x2004.
lw x2, 8(x1): If register x1 contains 0x1000, then “lw x2, 8(x1) will find the memory address 0x1008 and copy its contents into register x2.
-
computation instructions are related to what is stored in the address/reg
-
Simple computation structure
1)Load a,b,c with LW
2)Perform operation
3)Store result with SW
-
Register -> binary encoding: 5 bit, 32 registers(x0-x31)
-
example of fib logic: mv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17// x1 = n
// x2 = final result
bne x1, x0, start // branch if n!=0
li x2, 0
j end // pseudo instruction for jal x0, end
start:
addi x1, x1, -1 // n = n - 1
li x3, 0 // x = 0
li x2, 1 // y = 1 (you're returning y at the end, so use x2 to hold y)
loop:
bge x0, x1, end // stop loop if 0 >= n
addi x5, x3, x2 // tmp = x + y
mv x3, x2 // x = y (pseudo instruction for addi x3, x2, 0)
mv x2, x5 // y = tmp (pseudo instruction for addi x2, x5, 0)
addi x1, x1, -1 // n = n - 1
j loop // pseudo instruction for jal x0, loop
end:
Riscv lab¶
An architecture, or more sp ecically an instruction set architecture, is the interface b etween software and hardware. The instruction set architecture precisely sp ecies the resources of the machine and the way programs can access and use them.
-
Convert loop into branches and jumps
-
Condition at bottom
1
2
3
4
5
6
7
8
9
10
11
12
13maximum:
li a2, 0 // load immediate 0 into a2 (largest_so_far)
loop:
lw a3, 0(a0) // load word at [[address stored in a0] + 0] into a3 (w)
ble a3, a2, continue // skip next instruction if a3 (w) ≤ a2 (largest_so_far)
...
continue:
...
...
bnez a1, loop // go to top of lo op if a1 (n) 6= 0
done:
...
... -
Condition at top
1
2
3
4
5
6
7
8
9
10
11
12
13
14maximum:
li a2, 0 // load immediate 0 into a2 (largest_so_far)
loop:
beqz a1, done // go to <done> if a1 (n) = 0
lw a3, 0(a0) // load word at [[address stored in a0] + 0] into a3 (w)
ble a3, a2, continue // skip next instruction if a3 (w) ≤ a2 (largest_so_far)
...
continue:
...
...
j loop // go to top of lo op
done:
...
...
-
-
interacting with main memory: lw, sw
1
2lw rd, imm(rs) # we can load the word at address (n+imm) in memory into register rd.
sw rs2, imm(rs1) # we can save the content of rs2 into memory at address (content of rs1 + imm)