<<C++ Programming Excercises in CSAPP
Ps0¶
seems nice.
Sec 1¶
-
can’t call ++it when it == container.end(), otherwise segmentation fault.
-
Prefer ++i than i++
1
2
3
4
5i = 1;
j = ++i; //(i is 2, j is 2)
i = 1;
j = i++; // (i is 2, j is 1)
Ps1: a debugging memory allocator¶
Context:
- malloc is not perfect and can fail due to various reasons such as size was too big/memory is exhausted/…
- undefined behavior: any program that invokes undefined behavior has no meaning.
Syntax:
-
const
1
2
3
4// const pointer point to const member function which takes const pointer
// to a const int. The last const means this is a const member function
// (can't modify variables of the this instance)
const int* const myClassMethod(const int* const & param) const; -
type casting
1
2
3// internal metadata
std::unordered_map<uintptr_t, size_t> payload;
payload.insert({(uintptr_t)ptr, sz});
Problem:
- How to count the allocations?
- augmentation
- When will allocation fail?
- Stuck for two hours. I thought I could not modify funcation signature(return type). Therefore I overlooked the right direction.
?relation in heap max address and failed allocations.Answer: when malloc fails, base_alloc(malloc) will return a nullptr, which is the usual case.
- allocated address of heap: overlap with other regions
- Key: heap_first
- Answer: should set heap_min only once
Design:
- Basic version:
- use hash table(pointer value/address: size)
- bounded metadata: last N freed allocations, and set N = 10.
- should check size once updates data
- augmentation: use a struct
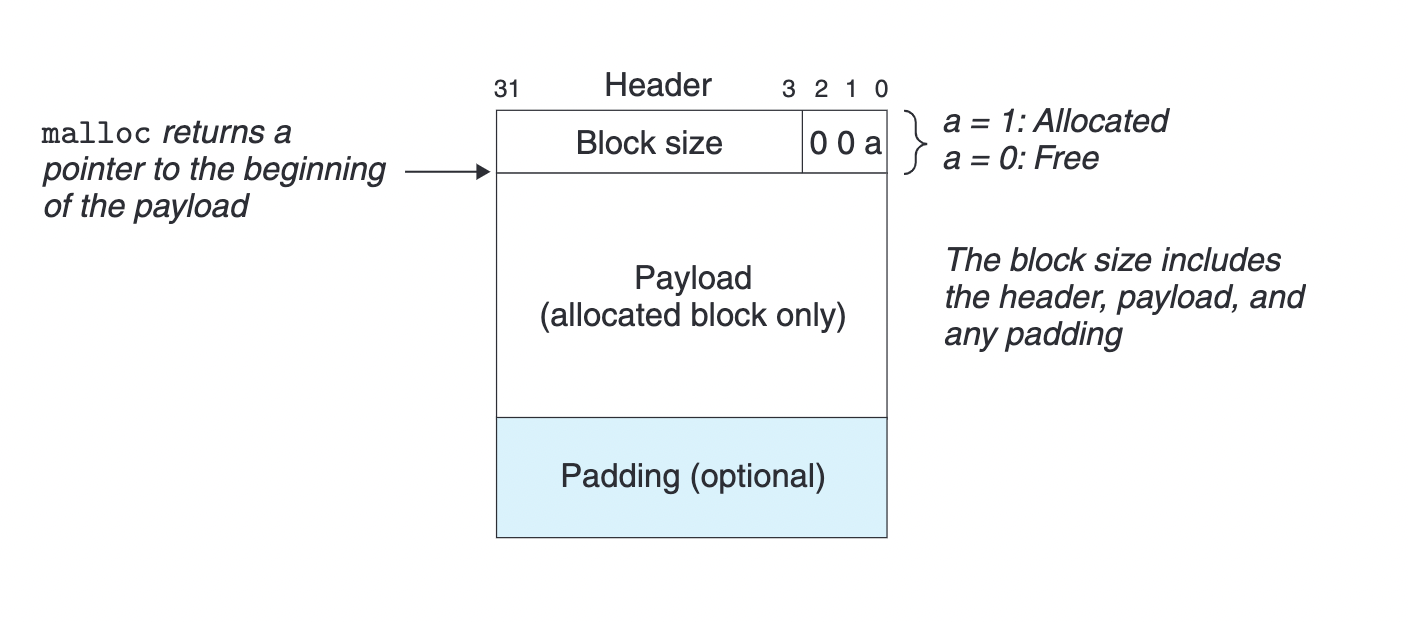
Problem:
- where to put metadata?
- global use
- How to bound metadata?
- First, what is bounded metadata?
- ex: a bounded amount of metadata about freed allocations, such as statistics or a list of the last N freed allocations.
- First, what is bounded metadata?